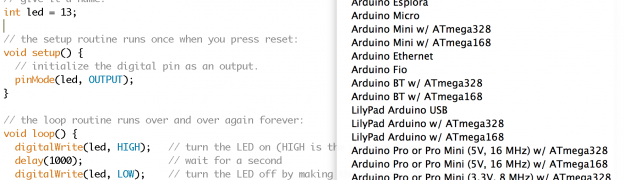
I had a bunch of ATmega 16A and ATtiny 85 microcontrollers lying around and I was trying to find a way to program them using Arduino code. There are two main reasons, why I wanted to use Arduino code. The first reason was to use the many built-in functions like digitalWrite
, digitalRead
etc. And the second reason was to use the various built-in and user contributed Arduino libraries.
Since the official Arduino supports more than 3 types of microcontrollers (Uno, Mega, Leonardo), I had a hunch that supporting other microcontrollers should be easy. With this in mind, I started digging into Arduino code. After referring to couple of files inside the hardware folder, I found a way by which you can easily add support for non-Arduino AVR microcontrollers.
pins_arduino.h file
The developers of Arduino, have cleanly separated out the pin definitions into a separate files. This allows you to easily add support for new non-Arduino AVR microcontrollers.
To add support for ATmega 16A, I just copied the Uno’s pin_arduino.h
file from hardware/arduino/variants/standard/
folder in Arduino installation directory and started modifying it.
In the pin_arduino.h
file, you need to change the following things.
- The number of digital pins
- The number of analog pins
- Analog pin mappings and const for Analog pins (actual values for A0, A1)
- Digital pin to PCICR mapping
- Pin to port (PORTA, PORTB etc) mapping
- Pin to timer mapping
Most of these are straight forward and you can get the correct values from the target AVR chips datasheet. You can refer to the pin mapping file for ATmega 16A that I created.
boards.txt file
The Arduino IDE (and also my Arduino makefile) get details about your board from a file named boards.txt
. This simple text file, has the details about your board and also instructs the Arduino IDE to use the proper parameters and fuses while compiling and uploading programs.
To let the Arduino IDE, know about our new microcontroller, we need to create a new boards.txt
file which has the following information about the microcontroller.
- Upload protocol
- Upload speed (baudrate)
- Upload fuses
- Clock frequency
- Maximum flash memory size
- Which variant (
pins_arduino.h
) file to use
Like the pins_arduino.h
file, most of these are straight forward and you can get most of these details from the target AVR chips datasheet. You can refer to the boards.txt
file, that I created for ATmega 16A.
Integration with Arduino IDE
Once we have created the pins_arduino.h
and boards.txt
file, we should then place them in a proper directory structure, which the Arduino IDE could understand.
First, create a directory called hardware
in your sketchbook directory. After that create a new directory inside it to keep all our files. I named it arduino-extra-cores
. Place the boards.txt
file inside this directory. Now create a directory named variants
and then create another directory with the name of your microcontroller. Place the pins_arduino.h
file inside this directory.
Once you have done this, the directory structure should look like this.
After doing this, restart your Arduino IDE and you should see the new entry that you created in the boards.txt
file under the Boards menu, like below.
To compile and upload Arduino programs to your new microcontroller, you just have to select the new entry from the Boards menu, everything else will be taken care by the Arduino IDE.
If you don’t have a programmer to connect your microcontroller, you can also use an Arduino as a programmer to upload your programs. To use serial monitor, with your microcontroller, you can also use an Arduino as a bridge.
I have also written an separate tutorial that explains how you can use Arduino as an ISP programmer to program ATMega 16/16A micro controllers.
Arduino Extra Cores
I have put together all of this into a github repo called Arduino Extra Cores. Right now it supports both ATmega 16 and ATmega 16A family of microcontrollers. I am planning to support for more microcontrollers soon.
Feel free to check it out and also to use it as your template. If you managed to port a new microcontroller, I would be happy to merge it into my Arduino Extra Cores repo.
Happy Hack’ing 🙂
Update: Kindly note that this works only in Arduino 1.0.x branch. The structure of hardware folder has changed in Arduino 1.5.x branch and I have not updated the core yet to support it. Will post an update, once I had support for Arduino 1.5.x as well.