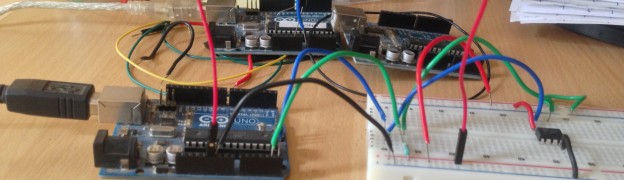
One of the main reasons why Arduino is very popular with beginners is that it is completely self-contained which makes it very easy to use. Once you have an Arduino board, all you have to do is to download the software and within minutes you can make your first led blink. The software has everything you need right from the IDE, to the compiler and even serial monitor to communicate with Arduino, all within one single package.
While the Arduino IDE is very good for beginners, you might start to feel its limitations, once you start working with it regularly. I use vim for everything and always wished writing Arduino sketches in vim.
I configured Arduino IDE, to use an external editor and started using vim to write code and then Arduino IDE to compile and upload. While this worked for sometime, I started to feel the need for a proper makefile so that I can do everything from vim itself.
Makefile for Arduino
My search landed me to a makefile created by Martin Oldfield. I started using it regularly and also contributed back my patches. Over a period of time, Martin lost interest in the project and now I took ownership of the project and maintain it.
The makefile is quite mature now and in most cases, you can use it to replace the Arduino IDE. There are still some corner cases, but I guess you may not hit them in your day to day use. Also the other advantage of using the makefile is that, you can even program directly using AVR C or assembly, which is not quite easy to do with the Arduino IDE.
Installation
The makefile requires the Arduino software to be installed. So if you have not installed it before, then you have to install it first.
There are three ways by which you can get the makefile
- Install through package
- Do a checkout from github
- Download zip file from github
Install through package
Packages are available for Debian, Ubuntu and FreeBSD. The package name is arduino-mk
. If you prefer to install a package, rather than checking out code from github, then you can install the package, if you are using Debian, Ubuntu or FreeBSD.
I also have plans to have a package for homebrew. I will post an update once the package is available for homebrew.
Do a checkout from github
The makefile is hosted in github. You can directly checkout from github. The advantage of this method is that it is every easy to get updates, since the project is currently under heavy development.
Download zip file from github
If you are not comfortable with git or don’t want to do a checkout, then you can also download the zip file from github.
Install dependencies
The Makefile delegates resetting the board to a short Perl program. You’ll need to install Device::SerialPort
and YAML
library.
On Debian or Ubuntu:
apt-get install libdevice-serialport-perl
apt-get install libyaml-perl
On Fedora:
yum install perl-Device-SerialPort
yum install perl-YAML
On Mac using MacPorts:
sudo port install p5-device-serialport
sudo port install p5-YAML
and use /opt/local/bin/perl5
instead of /usr/bin/perl
On other systems:
cpanm Device::SerialPort
cpanm YAML
Setup
Instead of copying the makefile to every sketch folder, you can just place the downloaded makefile separately in a common location and then create a small child makefile for every sketch.
Global variables
Once you have copied the makefile to a common location, or installed it through a package, you need to declare the following global variables. You can either declare them in your child makefile or set them as environmental variables.
ARDUINO_DIR
– Directory where Arduino is installedARDMK_DIR
– Directory where you have copied the makefileAVR_TOOLS_DIR
– Directory where avr tools are installed
I have the following setup in my ~/.bashrc
file
export ARDUINO_DIR=/home/sudar/apps/arduino-1.0.5
export ARDMK_DIR=/home/sudar/Dropbox/code/Arduino-Makefile
export AVR_TOOLS_DIR=/usr
Per sketch variables
After the global settings, you will need to specify the following variables for each sketch
BOARD_TAG
– The Arduino board that you are using. By defaultUno
is usedARDUINO_PORT
– The serial port where Arduino is connectedARDUINO_LIBS
– Space separated set of libraries that are used by your sketch
Usage
Compiling programs
To compile your programs you just have to invoke the command make
.
The output is pretty verbose and will list down the configurations that the makefile is using and from where it got the values.
All the build files will be created under a subdirectory in your sketch folder.
If there were any errors then they will be displayed with line numbers, which you can correct.
Uploading programs
To upload the compiled program to your Arduino, just plug your Arduino and run the command make upload
.
The program will be recompiled if needed and will be uploaded to your Arduino board.
Opening Serial monitor
The makefile can also be used to check the serial output from your Arduino. To do that just run the command make monitor
.
This command will open a serial connection to your Arduino using screen
.
The makefile tries to auto detect the baud rate. If it is not able to detect it properly, then you can manually set it using the variable MONITOR_BAUDRATE.
Advanced Usage
In addition to the above typical workflows, the makefile can also be used to do the following advanced stuff. I will write detailed guide for each of these use cases when I get some free time. Meanwhile you can also checkout some of the sample makefiles in the examples folder at github.
- Compiling plain AVR C programs
- Program using Arduino as ISP
- Generate assembly and symbol files
- Program using alternate Arduino core (like ATtiny or Arduino alternate cores)
Related projects
If you are using Vim as your editor for compiling Arduino programs then you might be interested in some of my following projects as well.
- Vim syntax files for Arduino
- Vim snippets for Arduino
- Arduino Extra Core – Additional cores for non Arduino AVR microcontrollers like ATmega 16
Do check them out as well ๐